Previous posts walk through how to run code on an esp32 emulator using QEMU and how to create virtualized sensors for esp32 emulator. This post…
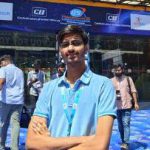
Posts published by “Aryan”
Aryan is an editor and TechKnow Geek at Club TechKnowHow! He loves sharing cool stuff about tech and code in a way that's easy to understand and apply. Connect with him on LinkedIn & Instagram for updates and fun techie chats.🚀
Last week Prakriti & I created BitFlash_Client which is now officially a part of the Arduino Library Registry. You can try out the library and…
Hosting websites on a windows server using the IIS – Internet Information Services is painful in 2024 for a new developer. The technology is so…